Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- CKA 기출문제
- golang
- CloudWatch
- APM
- 티스토리챌린지
- 오블완
- AI
- kotlin coroutine
- Linux
- aws
- Kubernetes
- Java
- AWS EKS
- 정보처리기사실기 기출문제
- Pinpoint
- 정보처리기사 실기 기출문제
- tucker의 go 언어 프로그래밍
- 코틀린 코루틴의 정석
- Spring
- kotlin
- PETERICA
- mysql 튜닝
- 기록으로 실력을 쌓자
- go
- docker
- kotlin querydsl
- minikube
- CKA
- 공부
- Elasticsearch
Archives
- Today
- Total
피터의 개발이야기
[Kotlin] List 사용법 정리 본문
반응형
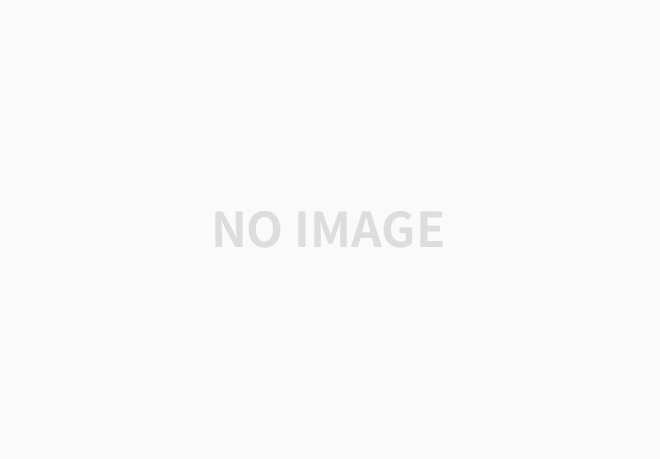
ㅁ 들어가며
ㅇ Kotlin에서 List는 순서가 있는 요소들의 컬렉션이다.
ㅇ 이 글에서는 List의 기본적인 사용법과 함께 자주 사용되는 연산들을 살펴본다.
ㅁ List 생성하기
val immutableList = listOf(1, 2, 3, 4, 5)
val mutableList = mutableListOf("a", "b", "c")
ㅁ List 요소 접근하기
val firstElement = immutableList[0]
val lastElement = immutableList.last()
val firstElement = immutableList[0] val lastElement = immutableList.last()ㅁ List 순회하기
for (item in immutableList) {
println(item)
}
immutableList.forEach { println(it) }
ㅁ 중복 요소 제거하기 (distinct)
val listWithDuplicates = listOf('a', 'b', 'c', 'a', 'c')
val distinctList = listWithDuplicates.distinct()
println(distinctList) // [a, b, c]
val list = listOf('a', 'A', 'b', 'B', 'c', 'A', 'a', 'C')
val distinctUppercaseList = list.distinctBy { it.uppercaseChar() }
println(distinctUppercaseList) // [a, b, c]
ㅇ 대소문자 구분 없이 중복 제거 방법
ㅁ List를 Set으로 변환하기
val list = listOf(1, 2, 3, 2, 1)
val set = list.toSet()
println(set) // [1, 2, 3]
ㅇ Set은 중복을 허용하지 않는 컬렉션이므로, List를 Set으로 변환하면 자동으로 중복이 제거된다.
ㅁ Set을 List로 변환하기
val set = setOf(1, 2, 3)
val list = set.toList()
println(list) // [1, 2, 3]
ㅁ List 필터링
val numbers = listOf(1, 2, 3, 4, 5, 6)
val evenNumbers = numbers.filter { it % 2 == 0 }
println(evenNumbers) // [2, 4, 6]
ㅁ List 변환 (map)
val numbers = listOf(1, 2, 3, 4, 5)
val squaredNumbers = numbers.map { it * it }
println(squaredNumbers) // [1, 4, 9, 16, 25]
ㅁ List 정렬하기
val unsortedList = listOf(3, 1, 4, 1, 5, 9, 2, 6, 5)
val sortedList = unsortedList.sorted()
println(sortedList) // [1, 1, 2, 3, 4, 5, 5, 6, 9]
ㅁ 마무리
ㅇ Kotlin의 List는 다양한 연산을 지원하여 데이터를 효율적으로 처리할 수 있게 해준다.
ㅇ 중복 제거, Set 변환, 필터링, 변환, 정렬 등의 기능을 활용하면 복잡한 데이터 처리 작업을 간단하게 수행할 수 있다.
ㅇ 또한 불변 List와 가변 List를 상황에 맞게 선택하여 사용함으로써 안전하고 유연한 코드를 작성할 수 있다.
ㅁ 함께 보면 좋은 사이트
ㅇ https://kotlinlang.org/api/latest/jvm/stdlib/kotlin.collections/-set/
ㅇ https://www.devkuma.com/docs/kotlin/list-distinct/
ㅇ https://www.devkuma.com/docs/kotlin/list-to-set/
ㅇ https://www.devkuma.com/docs/kotlin/set-to-list/
반응형
'Programming > Kotlin' 카테고리의 다른 글
[Kotlin] ManagementFactory을 이용한 JVM 모니터링 방법 (1) | 2024.07.14 |
---|---|
[Kotlin] kotlin 폴더 전체 삭제 방법 (0) | 2024.07.13 |
[Kotlin] File들을 코드별 폴더로 압축하기 (0) | 2024.07.11 |
[Kotlin] MutableList 기능 설명 (0) | 2024.07.10 |
[Kotlin] Map 다양한 사용법 (1) | 2024.07.09 |
Comments